Cache
Client put features in cache with their activation conditions (or alternative values for string/number features).
This means that (except for script features) client can compute feature value locally, without the need to perform an http call to remote Izanami. In this mode, feature activation conditions/alternative values are updated periodically in background.
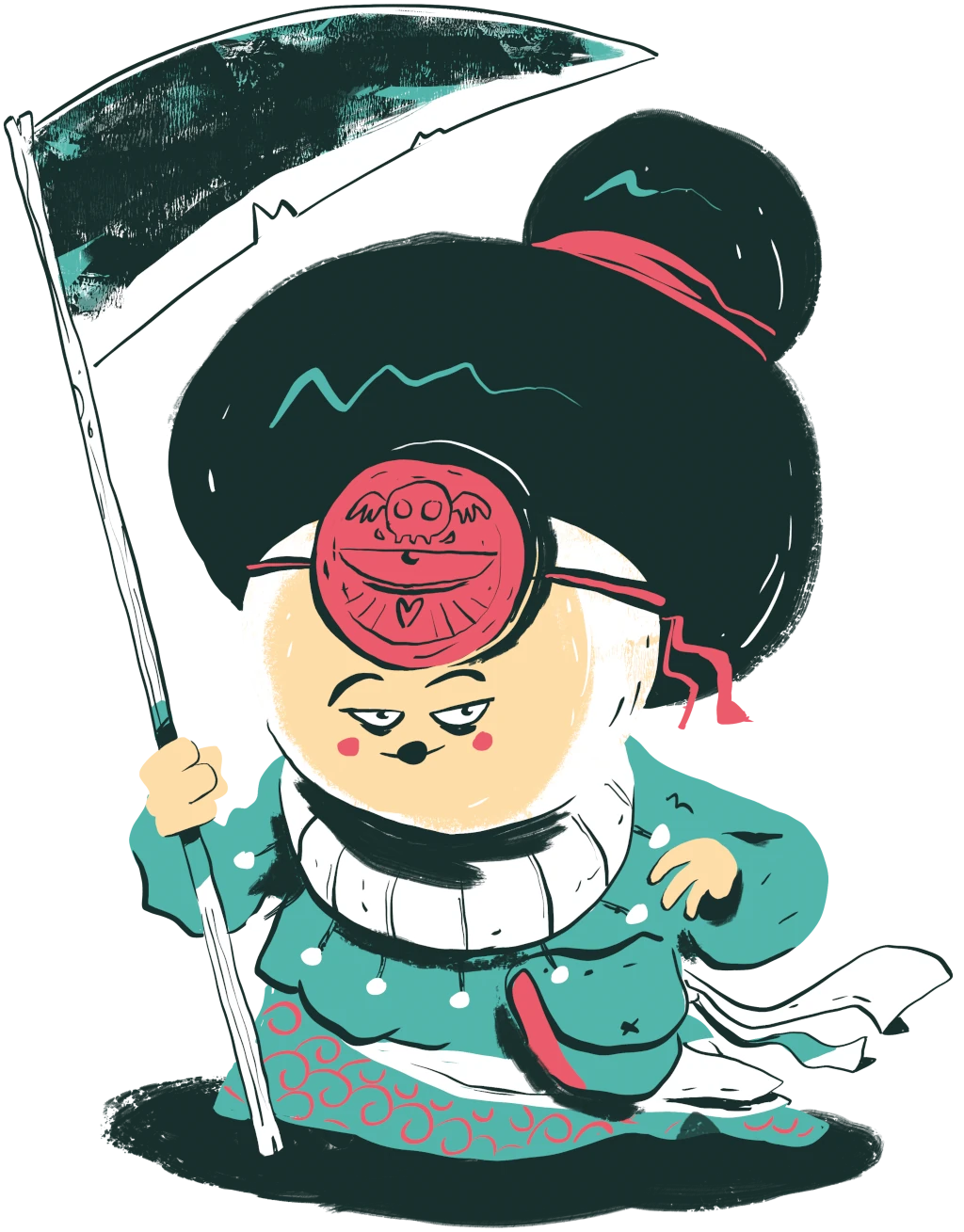
Script features can't be evaluated locally, since client does not embed a WASM runtime.
IzanamiClient client = IzanamiClient.newBuilder(
connectionInformation()
.withUrl(<REMOTE_IZANAMI_BASE_URL>/api)
.withClientId(<YOUR_KEY_CLIENT_ID>)
.withClientSecret(<YOUR_KEY_CLIENT_SECRET>)
).withCacheConfiguration(
FeatureCacheConfiguration
.newBuilder()
.enabled(true) // Enable caching feature activation conditions and local activation computation
.withRefreshInterval(Duration.ofMinutes(5L)) // Optional : how frequently cache will be refreshed
.build()
).build();
By default, cache is disabled and a query is sent to remote Izanami for each request.
When cache is active, it's always possible to skip it for some queries :
CompletableFuture<Boolean> result = client.booleanValue(
newSingleFeatureRequest(id)
.ignoreCache(true) // Ignore cache and perform request for this query
);
CompletableFuture<Boolean> another = client.booleanValue(
newFeatureRequest()
.withFeatures(<FEATURE_IDS>)
.ignoreCache(true) // Ignore cache and perform request for this query
);
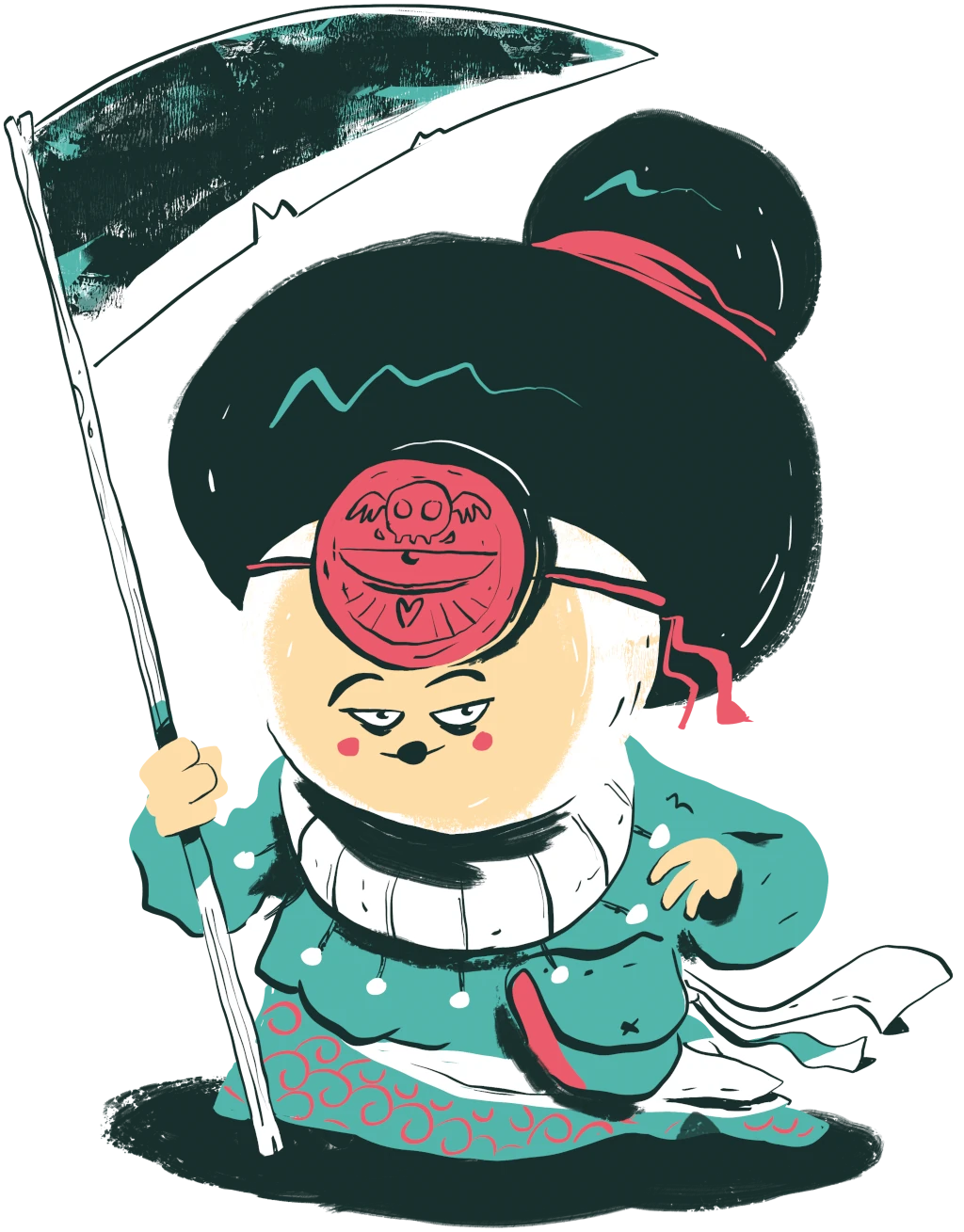
Ignoring cache this way will still update cache with retrieved activation conditions.